Password show and hide with eye button using javascript
In this article, we will learn how to implement the functionality of password show and hide with eye using JavaScript.
Nowadays, generally in the registration and login form, we need a password field. To make that password field attractive we can use the functionality of show and hide the password by clicking on the eye button.
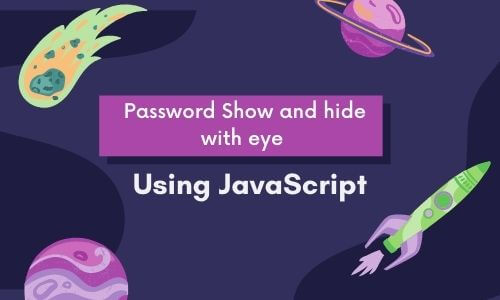
In this article, we used font awsome CDN to use the eyes icon. We also created a JavaScript function to make this happen, which is responding on the onClick() event.
<!DOCTYPE html> <html> <head> <title>Password Hide Show</title> </head> <!-- This is font awsome cdn to use eye icons --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> <body> <!-- This is Password field --> Password: <input type="password" id="passwordInputID" value="MyFakePassword"> <!-- This is eye button --> <span style="border:1px solid #ddd;padding:0 2px;"> <i id="eyeChangeId" class="fa fa-eye" onclick="eyeEnableOrDisable()"></i> </span> </body> <script type="text/javascript"> //Javascript function definition function eyeEnableOrDisable() { var x = document.getElementById("passwordInputID"); //getting the password field element var y = document.getElementById("eyeChangeId"); //getting the eye button element if (x.type === "password") { x.type = "text"; y.classList.remove("fa-eye"); y.classList.add("fa-eye-slash"); } else { x.type = "password"; y.classList.remove("fa-eye-slash"); y.classList.add("fa-eye"); } } </script> </html>
The main logic behind this is just to change the input type and changing the class name of the icon. It is very easy to implement password show and hide with eye button using JavaScript.
If you want to implement the same functionality using jQuery then follow our other article password show and hide using jQuery.